Post by farjana89 on Jun 8, 2024 8:19:05 GMT
Download File in English Language using Spring Boot
Are you looking to learn how to download a file using Spring Boot in English language for your next project? This article will guide you through the process step by step, covering all the essential aspects and providing you with the necessary code snippets to get you started.
Introduction
Spring Boot is a popular Java-based framework that simplifies the d thailand phone number evelopment of web applications. One common requirement in web development is the ability to allow users to download files from the server. In this article, we will focus on how to implement file downloads using Spring Boot, ensuring that your application is efficient and user-friendly.
How to Start with Spring Boot?
To begin with, make sure you have a basic understanding of Spring Boot and have it set up in your development environment. If you are new to Spring Boot, you can refer to the official documentation for a quick start guide. Once you have Spring Boot installed, you are ready to proceed with implementing the file download functionality.
File Download Implementation
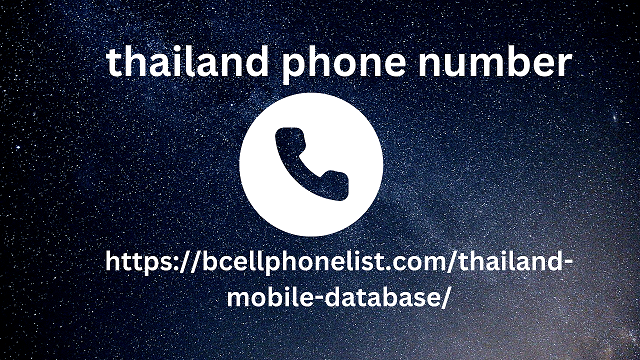
To enable file downloads in your Spring Boot application, you will need to create a controller that handles the download request. Below is a sample controller code that demonstrates how to achieve this:
@restcontroller
public class FileDownloadController {
@getmapping("/downloadFile")
public ResponseEntity<Resource> downloadFile() {
// Logic to fetch the file to be downloaded
Resource file = // Get the file resource
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + file.getFilename() + "\"")
.body(file);
}
}
In the above code snippet, we have created a REST controller that listens for GET requests on the '/downloadFile' endpoint. The controller fetches the desired file and returns it as a ResponseEntity with appropriate headers for file download.
Additional Considerations
Make sure to handle exceptions and error scenarios gracefully in your file download controller.
You can enhance the functionality by allowing users to download specific files based on parameters or criteria.
Consider implementing security measures to restrict access to certain files or authenticate users before allowing download.
Conclusion
In conclusion, implementing file downloads in your Spring Boot application is a straightforward process that can greatly enhance the user experience. By following the steps outlined in this article and adapting the code to suit your specific requirements, you can easily integrate file download functionality into your application.
Remember, the key to success lies in understanding the core concepts of Spring Boot and effectively utilizing its features to meet your development needs. So why wait? Start implementing file downloads in your Spring Boot application today!
Meta Description
Learn how to download files in English language using Spring Boot. Follow our simple guide for a seamless integration of file download functionality in your web application.
Are you looking to learn how to download a file using Spring Boot in English language for your next project? This article will guide you through the process step by step, covering all the essential aspects and providing you with the necessary code snippets to get you started.
Introduction
Spring Boot is a popular Java-based framework that simplifies the d thailand phone number evelopment of web applications. One common requirement in web development is the ability to allow users to download files from the server. In this article, we will focus on how to implement file downloads using Spring Boot, ensuring that your application is efficient and user-friendly.
How to Start with Spring Boot?
To begin with, make sure you have a basic understanding of Spring Boot and have it set up in your development environment. If you are new to Spring Boot, you can refer to the official documentation for a quick start guide. Once you have Spring Boot installed, you are ready to proceed with implementing the file download functionality.
File Download Implementation
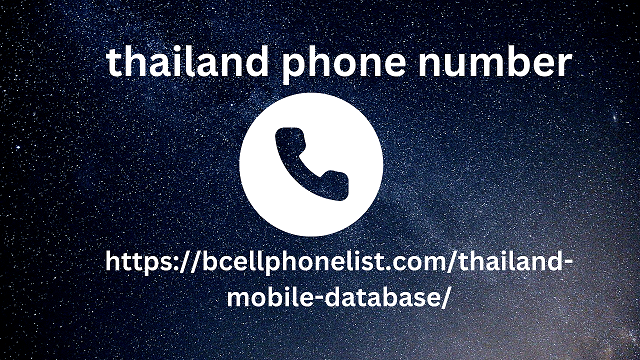
To enable file downloads in your Spring Boot application, you will need to create a controller that handles the download request. Below is a sample controller code that demonstrates how to achieve this:
@restcontroller
public class FileDownloadController {
@getmapping("/downloadFile")
public ResponseEntity<Resource> downloadFile() {
// Logic to fetch the file to be downloaded
Resource file = // Get the file resource
return ResponseEntity.ok()
.header(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=\"" + file.getFilename() + "\"")
.body(file);
}
}
In the above code snippet, we have created a REST controller that listens for GET requests on the '/downloadFile' endpoint. The controller fetches the desired file and returns it as a ResponseEntity with appropriate headers for file download.
Additional Considerations
Make sure to handle exceptions and error scenarios gracefully in your file download controller.
You can enhance the functionality by allowing users to download specific files based on parameters or criteria.
Consider implementing security measures to restrict access to certain files or authenticate users before allowing download.
Conclusion
In conclusion, implementing file downloads in your Spring Boot application is a straightforward process that can greatly enhance the user experience. By following the steps outlined in this article and adapting the code to suit your specific requirements, you can easily integrate file download functionality into your application.
Remember, the key to success lies in understanding the core concepts of Spring Boot and effectively utilizing its features to meet your development needs. So why wait? Start implementing file downloads in your Spring Boot application today!
Meta Description
Learn how to download files in English language using Spring Boot. Follow our simple guide for a seamless integration of file download functionality in your web application.